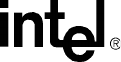
448 Voice API for Windows Operating Systems Library Reference — November 2003
li_attendant( ) — perform the actions of an automated attendant
#include <windows.h>
#include <stdlib.h>
#include <stdio.h>
#include <conio.h>
#include <process.h>
#include <srllib.h>
#include <dxxxlib.h>
#include "syntellect.h"
#define EXTENSION_LENGTH 2
#define EVENT_NAME "ExitEvent"
// define functions used for the hook
static int att_onhook(int dev); // optional
static int att_offhook(int dev); // optional
static BOOL att_mapextension(char *, char *); // obligatory !!
static int att_waitforrings(int dev, BOOL *bWaiting); // optional
int main (int argc, char *argv[])
{
HANDLE hEvent;
HANDLE hThread[2];
DX_ATTENDANT Att[2];
BOOL ret;
ZeroMemory(&Att, sizeof(Att));
// initialize structure for two thread
// thread 1 uses custom call back functions
// for telephony control
Att[0].nSize = sizeof(DX_ATTENDANT);
strcpy(Att[0].szDevName, "dxxxB1C1");
Att[0].pfnDisconnectCall = (PFUNC) att_onhook;
Att[0].pfnAnswerCall = (PFUNC) att_offhook;
Att[0].pfnExtensionMap = (PMAPFUNC) att_mapextension;
Att[0].pfnWaitForRings = (PWAITFUNC) att_waitforrings;
strcpy(Att[0].szEventName, EVENT_NAME);
Att[0].nExtensionLength = EXTENSION_LENGTH;
Att[0].nDialStringLength = EXTENSION_LENGTH+10;
Att[0].nTimeOut = 5;
// thread 2 uses built-in functions
// for telephony control
Att[1].nSize = sizeof(DX_ATTENDANT);
strcpy(Att[1].szDevName , "dxxxB1C2");
Att[1].pfnDisconnectCall = (PFUNC) NULL;
Att[1].pfnAnswerCall = (PFUNC) NULL;
Att[1].pfnExtensionMap = (PMAPFUNC) att_mapextension;
Att[1].pfnWaitForRings = (PWAITFUNC) NULL;
strcpy(Att[1].szEventName , EVENT_NAME);
Att[1].nExtensionLength = EXTENSION_LENGTH;
Att[1].nDialStringLength = EXTENSION_LENGTH+10;
Att[1].nTimeOut = 5;
// create the named event
if ((hEvent = CreateEvent(
NULL, // no security attributes
TRUE, //FALSE, // not a manual-reset event
FALSE, // initial state is not signaled
EVENT_NAME // object name
)) == (HANDLE) NULL)
return (-1);