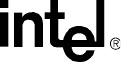
Voice API for Windows Operating Systems Library Reference — November 2003 303
play recorded voice data — dx_play( )
/* Play a voice file. Terminate on receiving 4 digits or at end of file*/
#include <fcntl.h>
#include <srllib.h>
#include <dxxxlib.h>
#include <windows.h>
main()
{
int chdev;
DX_IOTT iott;
DV_TPT tpt;
DV_DIGIT dig;
.
.
/* Open the device using dx_open( ). Get channel device descriptor in
* chdev.
*/
if ((chdev = dx_open("dxxxB1C1",NULL)) == -1) {
/* process error */
}
/* set up DX_IOTT */
iott.io_type = IO_DEV|IO_EOT;
iott.io_bufp = 0;
iott.io_offset = 0;
iott.io_length = -1; /* play till end of file */
if((iott.io_fhandle = dx_fileopen("prompt.vox", O_RDONLY|O_BINARY))
== -1) {
/* process error */
}
/* set up DV_TPT */
dx_clrtpt(&tpt,1);
tpt.tp_type = IO_EOT; /* only entry in the table */
tpt.tp_termno = DX_MAXDTMF; /* Maximum digits */
tpt.tp_length = 4; /* terminate on four digits */
tpt.tp_flags = TF_MAXDTMF; /* Use the default flags */
/* clear previously entered digits */
if (dx_clrdigbuf(chdev) == -1) {
/* process error */
}
/* Now play the file */
if (
dx_play(chdev,&iott,&tpt,EV_SYNC)
== -1) {
/* process error */
}
/* get digit using dx_getdig( ) and continue processing. */
.
.
}
!
!!
! Example 2
This example illustrates how to use dx_play( ) in asynchronous mode.
#include <stdio.h>
#include <srllib.h>
#include <dxxxlib.h>
#include <windows.h>